The field is a GUID because that is what is in the Sitecore value field, for the category field. I imagine its a droplist or a multilist. So what you need to do is create a new ComputedIndexField to turn that GUID into a name.
I am going to break the code up into Multilist field and a droplist field. They are a little different.
Code For Multilist Field
In the code below, I am taking the MultiList field and getting the selected items, find the Display Names of the items, adding them a list of strings that is my return. I return the list of strings to the indexer, which with then show me names in my facets.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
namespace Sitecore.Feature.Article.Search.ComputedFields
{
using Sitecore.ContentSearch;
using Sitecore.ContentSearch.ComputedFields;
using Sitecore.ContentSearch.Utilities;
using Sitecore.Data;
using Sitecore.Data.Fields;
using Sitecore.Foundation.SitecoreExtensions.Extensions;
public class TagNameComputedField : IComputedIndexField
{
public object ComputeFieldValue(IIndexable indexable)
{
var item = (SitecoreIndexableItem)indexable;
if (item == null)
{
return null;
}
if (!item.Item.IsDerived(Template.Article.ID)) return null;
var db = item.Item.Database;
MultilistField tags = item.Item.Fields[Template.Article.Fields.Tags];
if (tags == null || tags.TargetIDs.Length == 0) return null;
var list = new List<string>();
this.GetTags(tags.TargetIDs, list, db);
return list;
}
public void GetTags(ID[] tags, List<string> list, Database db)
{
tags.ForEach(tag => list.Add(db.GetItem(tag)?.DisplayName));
}
public string FieldName { get; set; }
public string ReturnType { get; set; }
}
}
The Config For Multilist Field
This is where we tie it together. I am adding my new tag_name
index field to my default Lucene indexes. The most important this here is the use os UNTOKENIZED
in the indexType. This makes Lucene save the whole name to the index in one piece. Not breaking up into terms. So My brown dog
goes into the index as a whole and not three different words.
<?xml version="1.0" encoding="utf-8"?>
<configuration xmlns:patch="http://www.sitecore.net/xmlconfig/" xmlns:set="http://www.sitecore.net/xmlconfig/set/">
<sitecore>
<contentSearch>
<indexConfigurations>
<defaultLuceneIndexConfiguration type="Sitecore.ContentSearch.LuceneProvider.LuceneIndexConfiguration, Sitecore.ContentSearch.LuceneProvider">
<fieldMap type="Sitecore.ContentSearch.FieldMap, Sitecore.ContentSearch">
<fieldNames hint="raw:AddFieldByFieldName">
<field fieldName="tag_name" storageType="YES" indexType="UNTOKENIZED" vectorType="NO" boost="1f" type="System.Collections.Generic.List`1[[System.String, mscorlib]]" settingType="Sitecore.ContentSearch.LuceneProvider.LuceneSearchFieldConfiguration, Sitecore.ContentSearch.LuceneProvider">
<Analyzer type="Sitecore.ContentSearch.LuceneProvider.Analyzers.LowerCaseKeywordAnalyzer, Sitecore.ContentSearch.LuceneProvider" />
</field>
</fieldNames>
</fieldMap>
<documentOptions type="Sitecore.ContentSearch.LuceneProvider.LuceneDocumentBuilderOptions, Sitecore.ContentSearch.LuceneProvider">
<fields hint="raw:AddComputedIndexField">
<field fieldName="tag_name" storageType="no" indexType="untokenized">Sitecore.Feature.Article.Search.ComputedFields.TagNameComputedField, Sitecore.Feature.Article</field>
</fields>
</documentOptions>
</defaultLuceneIndexConfiguration>
</indexConfigurations>
</contentSearch>
</sitecore>
</configuration>
Sitecore facet
Now create your facet again, but use the index field name of tag_name. It will show you tags with real names and not GUIDs.
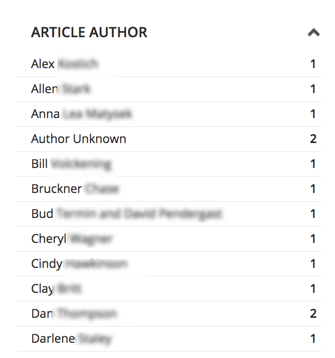
For a droplist field, we don't want a list of strings. We just need a single string of the name. So here is the code and config for a single item in a droplist.
The Code For Droplist Field
Here we just want to return a single string to the index.
namespace Sitecore.Feature.Article.Search.ComputedFields
{
using Sitecore.ContentSearch;
using Sitecore.ContentSearch.ComputedFields;
using Sitecore.Data;
using Sitecore.Data.Fields;
using Sitecore.Diagnostics;
using Sitecore.Foundation.SitecoreExtensions.Extensions;
public class AuthorNameComputedField : IComputedIndexField
{
public object ComputeFieldValue(IIndexable indexable)
{
var item = (SitecoreIndexableItem)indexable;
if (item == null)
{
return null;
}
if (!item.Item.IsDerived(Template.Article.ID)) return null;
var db = item.Item.Database;
var itemId = item.Item.Fields[Template.Article.Fields.Author].Value;
if (itemId == null || !ID.IsID(itemId)) return null;
var author = db.GetItem(new ID(itemId));
Log.Info($"Saving the index author value {author.DisplayName}", "indexing");
return author.DisplayName;
}
public string FieldName { get; set; }
public string ReturnType { get; set; }
}
}
The Config For Droplist Field
<?xml version="1.0" encoding="utf-8"?>
<configuration xmlns:patch="http://www.sitecore.net/xmlconfig/" xmlns:set="http://www.sitecore.net/xmlconfig/set/">
<sitecore>
<contentSearch>
<indexConfigurations>
<defaultLuceneIndexConfiguration type="Sitecore.ContentSearch.LuceneProvider.LuceneIndexConfiguration, Sitecore.ContentSearch.LuceneProvider">
<fieldMap type="Sitecore.ContentSearch.FieldMap, Sitecore.ContentSearch">
<fieldNames hint="raw:AddFieldByFieldName">
<field fieldName="author_name" storageType="YES" indexType="UNTOKENIZED" vectorType="NO" type="System.String" settingType="Sitecore.ContentSearch.LuceneProvider.LuceneSearchFieldConfiguration, Sitecore.ContentSearch.LuceneProvider" />
</fieldNames>
</fieldMap>
<documentOptions type="Sitecore.ContentSearch.LuceneProvider.LuceneDocumentBuilderOptions, Sitecore.ContentSearch.LuceneProvider">
<fields hint="raw:AddComputedIndexField">
<field fieldName="author_name" storageType="no" indexType="untokenized">Sitecore.Feature.Article.Search.ComputedFields.AuthorNameComputedField, Sitecore.Feature.Article</field>
</fields>
</documentOptions>
</defaultLuceneIndexConfiguration>
</indexConfigurations>
</contentSearch>
</sitecore>
</configuration>